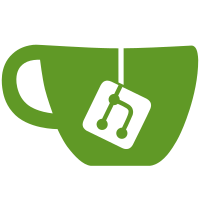
This commit moves the media scanner out of the db module and into a new module, media. We also change the --env parameter to --root, which takes a path to a directory where the default configuration and the transcoded media cache will live.
40 lines
1.1 KiB
Python
40 lines
1.1 KiB
Python
from hashlib import blake2b
|
|
from hmac import compare_digest
|
|
from typing import List
|
|
import os
|
|
|
|
|
|
def encode(args: List, uri: str) -> str:
|
|
"""
|
|
Encode a request and cryptographically sign it. This serves two purposes:
|
|
First, it enables the handler that receives the request to verify that the
|
|
request was meant for it, preventing various routing and relay-induced bugs.
|
|
Second, it ensures the request wasn't corrupted or tampered with during
|
|
transmission.
|
|
|
|
Args:
|
|
args (List): a list of parameters to pass along with the request.
|
|
uri (String): the URI of the intended handler for the request.
|
|
|
|
Returns:
|
|
String: A cryptographically signed request.
|
|
"""
|
|
return sign(uri + '\0' + '\0'.join(args))
|
|
|
|
|
|
def sign(request):
|
|
"""
|
|
Sign a request with a cryptographic hash. Returns the hex digest.
|
|
"""
|
|
h = blake2b(digest_size=16, key=bytes(os.environ['SECRET_KEY'].encode()))
|
|
h.update(request.encode())
|
|
return h.hexdigest()
|
|
|
|
|
|
def verify(request, digest):
|
|
return compare_digest(request, digest)
|
|
|
|
|
|
def url():
|
|
return f"http://{os.environ['HOST']}:{os.environ['PORT']}"
|